To begin using Facebook APIs, one should start a
After a successful initialization of the
Now for the fun stuff. I used the Facebook APIs asynchronously due to
the latency that may be noticed due to network issues. It goes like
that...
For publishing, the main function used here is
Source code :
FacebookService
giving it the application API (refer to Background
section). Also, according to the application, some user permissions may
be needed to be acquired. To get a list of permissions, you can go to http://developers.facebook.com/docs/authentication/permissions. // The application key of the Facebook application used
fbService.ApplicationKey = "";
// Add all needed permissions
List<Enums.ExtendedPermissions> perms = new List<Enums.ExtendedPermissions>
{
Enums.ExtendedPermissions.none
};
fbService.ConnectToFacebook(perms);
Important note: Some permissions are not yet listed in the current SDK, so I sometimes need to add them in the Enums.ExtendedPermissions
class and re-compile the source. After a successful initialization of the
FacebookService
,
running the application now shall result in the following dialog that
is used to start a session between a user and Facebook servers.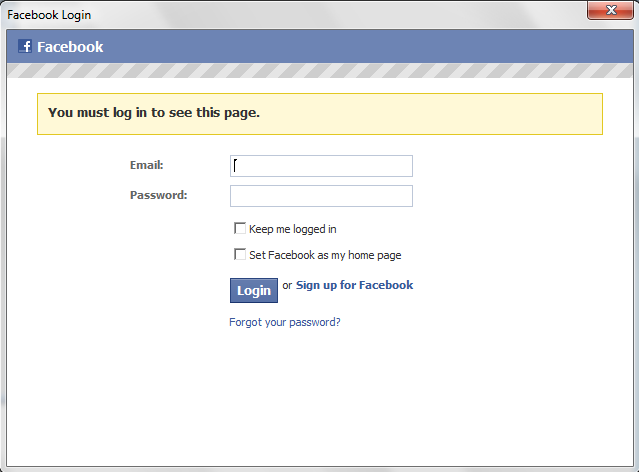
For publishing, the main function used here is
Stream.PublishAsync
.
All I have done is write a neat interface to use this function along
with the required callback (a function that is called when the
asynchronous result is received successfully).Source code :
public void PublishToAFriendWall()
{
try
{
attachment att = new attachment
{
// Name of link
name = "",
// URL of link
href = "",
caption = "",
media = new List<attachment_media>()
};
attachment_media_image attMEd = new attachment_media_image
{
// Image source
src = "",
// URL to go to if clicked
href = ""
};
att.media.Add(attMEd);
action_link a = new action_link
{
text = "What's this",
//URL to go to if clicked
href = ""
};
IList<action_link> tempA = new List<action_link> { a };
// Use the typed friend UID to publish the typed message
fbService.Stream.PublishAsync(friendWallTextBox.Text, att,
tempA, uidTextBox.Text, 0, PublishAsyncCompleted, null);
}
catch (Exception)
{
}
}
private static void PublishAsyncCompleted(string result, Object state,
FacebookExeption e)
{
}
For the other functionalities, the Facebook APIs used are: Photos.CreateAlbumAsync
for creating albums asynchronouslyPhotos.UploadAsync
for uploading photos asynchronouslyPhotos.AddTag
for tagging photos- And for the adding issue, I used the Internet Explorer DLL file (refer to Background section) to pop up a controlled Internet Explorer window showing the page http://www.facebook.com/addfriend.php?id= appended to it the uid of the user to add as a friend.
No comments:
Post a Comment